2020. 2. 10. 10:12ㆍ카테고리 없음
. Introduction This article is the first part of the series on Getting Started with Entity Framework Core. In this post, we will build an ASP.NET Core MVC application that performs basic data access using Entity Framework Core. We will explore the database-first approach and see how models are created from an existing database. We will also take a look at how classes become the link between the database and ASP.NET Core MVC Controller. Background In Software Development world, most applications require data store or database. So we all need a code to read/write our data stored in database or data store.
Creating and maintaining code for database is a tedious work and it is a real challenge for us. That's where Object Relational Mappers like Entity Framework comes into place. What is an Object Relational Mapper? An ORM enables developers to create data access applications by programming against a conceptual application model instead of programming directly against a relational storage schema. The goal is to decrease the amount of code and maintenance required for data-oriented applications. ORM like Entity Framework Core provides the following benefits:.
Modifying data via the DbContext The approach that you adopt to modifying entities depends on whether the context is currently tracking the entity being modified or not. In the following example, the entity is obtained by the context, so the context begins tracking it immediately. Entity framework core (v2.0) allows separating DB schema into multiple projects where one project holds one or more DB contexts. Sample solution will use the following tables in two schemas: Users.User.
Applications can work in terms of a more application-centric conceptual model, including types with inheritance, complex members, and relationships. Applications are freed from hard-coded dependencies on a particular data engine or storage schema. Mappings between the conceptual model and the storage-specific schema can change without changing the application code. Developers can work with a consistent application object model that can be mapped to various storage schemas, possibly implemented in different database management systems.
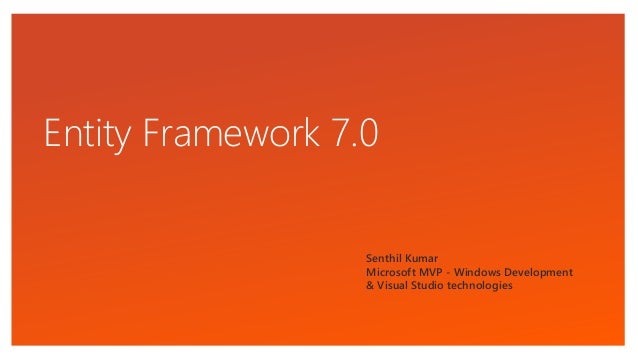
Multiple conceptual models can be mapped to a single storage schema. Language-integrated query (LINQ) support provides compile-time syntax validation for queries against a conceptual model. What is Entity Framework Core? According to the official: Entity Framework (EF) Core is a lightweight, extensible, and cross-platform version of the popular Entity Framework data access technology. EF Core is an object-relational mapper (O/RM) that enables.NET developers to work with a database using.NET objects. It eliminates the need for most of the data-access code that developers usually need to write.
To get more information about EF Core, I would recommend you to visit the official documentation here: Design WorkFlows Just like any other ORM, there are two main design workflows that is supported by Entity Framework Core: The Code-First approach which is you create your classes (POCO Entities) and generate a new database out from it. The Database-First approach allows you to use an existing database and generate classes based on your database schema. In the part let's explore Database-First approach first. If you are still confuse about the difference and advantages between the two, don't worry as we will be covering that in much detail in the upcoming part of the series. If you're ready to explore EF Core database-first development and get your hands dirty, then let's get started!
Database Creation In this walkthrough, we will just be creating a single database table that houses some simple table properties for simplicity. Quote: Notes: 1. It is always recommended to install the latest stable version of each package to avoid unexpected errors during development. If you are getting this error “Unable to resolve 'Microsoft.EntityFrameworkCore.Tools (= 1.1.2)' for '.NETCoreApp,Version=v1.1'” when building the project, then just restart Visual Studio 2017. When it’s done restoring all the required packages, you should be able to see them added to your project dependencies as shown in the figure below: Figure 10: Entity Framework Core Packages Restored For details about the new features in Entity Framework Core, check out the references section at the end of this article. Creating Entity Models from Existing Database Now, it’s time for us to create the Entity Framework models based on our existing database that we have just created earlier.
As of this time of writing, there are two ways to generate models from our existing database (a.k.a reverse engineer). Option 1: Using Package Manager Console. Go to Tools – NuGet Package Manager – Package Manager Console. And then run the following command below to create a model from the existing database: Scaffold-DbContext ' Server=SSAI-L0028-HP SQLEXPRESS;Database=EFCoreDBFirstDemo;TrustedConnection=True;' Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models/DB The Server attribute above is the SQL server instance name. You can find it in SQL Management Studio by right clicking on your database. For this example, the server name is “SSAI-L0028-HP SQLEXPRESS”. The Database attribute is your database name.
In this case, the database name is “EFCoreDBFirstDemo”. The –OutputDir attribute allows you to specify the location of the files generated. In this case, we’ve set it to Models/DB. Option 2: Using Command Window If for some unknown reason Option 1 will not work for you, try the following instead:. Go to the root folder of your application. In this case the “DBFirstDevelopment”. Do a Shift + Right Click and select “Open command window here”.
Then run the following script: dotnet ef dbcontext scaffold ' Server=SSAI-L0028-HP SQLEXPRESS;Database=EFCoreDBFirstDemo;TrustedConnection=True;' Microsoft.EntityFrameworkCore.SqlServer -output-dir Models/DB The script above is quite similar to the script that we used in Option 1, except we use the command dotnet ef dbcontext scaffold and -output-dir to set the target location of the scaffold files. A code monkey who loves to drink beer, play guitar and listen to music. My exploration into programming began at the age of 15;Turbo PASCAL, C, C, JAVA, VB6, Action Scripts and a variety of other equally obscure acronyms, mainly as a hobby. After several detours, I am here today on the VB.NET to C# channel. I now work on ASP.NET/Core + C# + MSSQL/Postgres + EF + Web API + JavaScripts + AJAX + Xamarin, which go together like coffee crumble ice cream. 9-time Microsoft MVP, 3-time C# Corner MVP, CodeProject MVP, Microsoft Influencer, Dzone MVB and a regular contributor at CodeAsp.Net in which I also moderate, C# Corner, AspSnippets, Xamarin but more often at the official Microsoft ASP.NET community site where I became one of the All-Time Top Answerer with ALL-STAR recognition level (the highest attainable level). My main interests include technologies, travel, beaches, mountains, paintings, arts, supernatural, ghosts, angels, vampires, mythology, mysteries, para-sciences, scifi,music, guitar, cars and motorbikes.
Mayupamu 20-Jul-18 8:31 20-Jul-18 8:31 Thanks for sharing. I followed all your steps and I am getting following error when trying to generate view from controller Please help me Method not found: 'Void Microsoft.EntityFrameworkCore.Design.Internal.DbContextOperations.ctor(Microsoft.EntityFrameworkCore.Design.Internal.IOperationReporter, System.Reflection.Assembly, System.Reflection.Assembly)'. At Microsoft.VisualStudio.Web.CodeGeneration.ActionInvoker.b60 at Microsoft.Extensions.CommandLineUtils.CommandLineApplication.Execute(String args) at Microsoft.VisualStudio.Web.CodeGeneration.ActionInvoker.Execute(String args) at Microsoft.VisualStudio.Web.CodeGeneration.CodeGenCommand.Execute(String args). Hi, sorry for the late answer, I hope you are still interested on this subject. I was trying to build the project on.NET Core 2 and I got the same problem as you did. The solution is to right-click on the Controllers folder, then Add, and choose New Scaffolded Item.
From the menu list, select MVC Controller with views, using Entity Framework. This will get you to the window menu described in the article, the one where you have to select the Model class, Data context class etc. And generate the Views.
Member 10579971 30-May-18 17:48 30-May-18 17:48 Hello, I am new to Entity Framework but I really enjoy learning from your article! I have some question regarding the step process, I know there is a code-first and database-first approach in entity framework, but here in step 01 you provided the sql script for creating the database, and then created domain object class with configuration(Entity Layer) in entity framework, I got confused here is that is which approach are you using here? Do I need to run your sql script first or maybe not because your Entity Layer looks exactly like code-first approach which should automatically created the same database structure, am I correct?
So if I have an existed database structure already, can I still use code-first like defining the Entity Layer in my project or I have to follow database-first approach and generate entity layer by using command like 'Scaffold-DbContext' as indicated in Please help me understand more about it! Thanks very much to everyone who can share your knowledge! 1) In that case you need to make changes in database and then make changes for C# classes without using scaffolding commands 2) I don't like use DbSet properties, but there isn't any difference between on the fly DbSet or DbSet properties 3) Have you review GetOrdersAsync method in SalesRepository class? 4) Include/ThenInclude load all properties for navigation properties and make the relations using defined primary keys, Linq-Sql join allows to create relations with or without defined primary keys.
I hope this helps. Anyway, if you have more questions don't hesitate to contact me. Hi HHerzl, it's me again.sorry I am reading code slowly and I really learned a lot from it, finally I still have couple of questions regarding the learning process, and I would really appreciate if you could help me figure out whenever you are available! 1) In Store.Core EntityLayer - I see each of your data entity model has a Byte Timestamp, but since it also have CreationDateTime and LastUpdateDateTime, just curious why would we need this Timestamp field for?
2) In Store.Core Contracts and Repository - I see you have an interface IRepository which contains the definition of CommitChange and CommitChangeAsync, however, in your Repository abstract class, you are not implement this IRepository there, but still implment those two methods, public abstract class Repository is that something you missed to implement the IRepository or the Repository abstract class should not implement IRepository, if so then why we need the IRepository? Hi 1) That's concurrency token for EF Core 2) IRepository interface is implemented by final repositories classes (SalesRepository, ProductionRepository) 3) I have no tested change log for child objects, let me check please 4) At this moment, there isn't security implementation for API.
Entity Framework Core Vs 6
Later IUser interface will replace with another security schema, take a look on code improvements please 5) In order to save in OrderDetail we need to set OrderID value, value for OrderID is set once AddOrderAsync is called Let me know if this is useful Regards. Hi HHerzl, Thank you very much for your reply and it was very helpful! For No.5, I understand the process to set OrderID in OrderDetail, but by looking at the documentation from Microsoft, it looks like we can directly save the related data entity with base data entity by calling SaveChange(in your example should be the CommitChangesAsync), that's why I am curious that in your approach, is there any benefit or usage by making it as a Database Transaction?
I guess it's for ' Rollback' usage that if something wrong in the middle of the whole process, whether saving Order/OrderDetail/Product, it can be rollback in the exception catch. As always, thanks a million for your guide and tutorial!